After learning the basics in our previous tutorials about if-else statements, switch-case statements, etc, it’s time to create functions in shell scripts. Functions help us divide scripts into different sections and make the code more portable as well as reusable.
Defining Functions in Shell Scripts
The basic syntax for defining a function is just the function name followed by round brackets. See the example below.
function_name(){
code and variables to work with
}
Now that you know how to define functions, let’s go about creating some real functions. You might have a question right now: How do you pass parameters to your functions?
Bash functions are already prepared to accept arguments. They’re supposed to be passed in a similar fashion to how we pass shell arguments. Let’s see a few examples to understand this.
Passing Arguments to Bash Functions
#!/bin/bash
new_function() {
echo "Hi! How's it going?"
echo "I was invoked with $1 $2"
}
new_function HowLinux Example
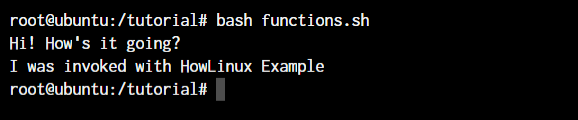
As you can see, I invoked the function with “Howlinux Example” and that was automatically passed on to the function. Now I can pass unlimited functions but since the function doesn’t know what to do with the rest of the arguments, those arguments won’t be printed out.
How do we print out any number of arguments then? We’ll simply replace the $1 $2 with $@ and it will print out all the arguments that are passed to it. Simple?
Working With Function Return Values
Alright, now not only do we need functions that perform tasks on given arguments, but we also need to return the values back and figure out how to use them.
This is very easy too. For this, we’ll need the $? operator which stores the return value of the command prior to this operator.
#!/bin/bash
new_function() {
echo "Hi! How's it going?"
echo "I was invoked with $@"
return 1
}
new_function HowLinux Example
echo $?
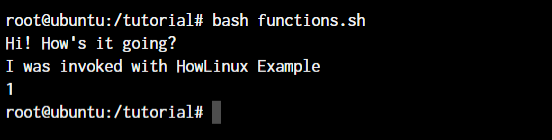
As you can see, the return value of 1 was captured and printed. Another way is to assign the return value to a variable (which by default is globally accessible across the code) and then just use the variable across the script.
Calling Functions from other Functions in Shell Scripts
Similar to how we called the function from outside, we can call another function from within our function. In the below example, I’m calling the second function (function2) with the argument that was passed to function1 and adding 1 to it. Learn more about how to perform operations on variables.
#!/bin/bash
function1() {
echo "this is function 1 $1"
function2 $(($1+1))
}
function2() {
echo "This is function 2 $1"
}
function1 5

Variable Scope Within Bash Functions
Most programming languages offer us the functionality of variable scopes. Just like it is with many languages, the default declaration of a variable is accessible globally. To make a variable accessible only within functions many programming and scripting languages provide with scope keywords. Let’s have a look at the scope keyword for bash.
#!/bin/bash
A="This is global value for A"
function1() {
local A="This is local value for A"
B="This is global and local value for B"
echo $A
echo $B
}
function1
echo $A
echo $B
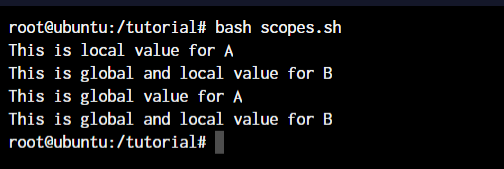
With the use of the “local” keyword in the above script, the local value of “A” is completely different from the global value. But the variable name is the same.
Conclusion
It should be easy for you to now move to some advanced scripting for yourself. Create some scripts for yourself. Make multiple functions, and maybe even try creating a loop for your function using functions that call each other. It would turn out to be a fun project and you will learn a lot in the process. Hope this tutorial has been useful to you.