In this article of the shell scripting tutorial series, we’ll learn how to create and use variables in shell scripts. We’ll walk through the different variables that we can make use of that are provided by the system for the Bourne Shell and how to create custom variables to use throughout a shell script.
Variables in shell scripts are used for storing values that you need to use frequently throughout a script. The variables can be set by either user input, shell arguments, or can be embedded directly into the shell. There are no fixed reasons for you to use either of the options except for what you want the script for and how you believe your users will make use of the scripts.
What are Variables in Shell Scripts?
If you’re familiar with any programming language, you have come across or even used variables before. Similar to the variables in other languages, variables in shell scripts serve the same purpose of storing data for use later.
The one difference you’ll observe in shell script variables compared to typed languages is that you do not need to declare the variable type when setting them. The system automatically understands the type of the variable being used.
How to Use Variables in a Shell Script?
Let’s begin with the basics. How do we set a variable? It’s simple. We’ll make use of the assignment operator “=” to assign values to a variable just like it is the case with pretty much all the programming languages.
Assigning Values To Variables
We’ll try to assign the values to a variable, one without spaces, second with spaces. Let’s see the outputs.
root@ubuntu:~# name=HowLinux
root@ubuntu:~# name = HowLinux
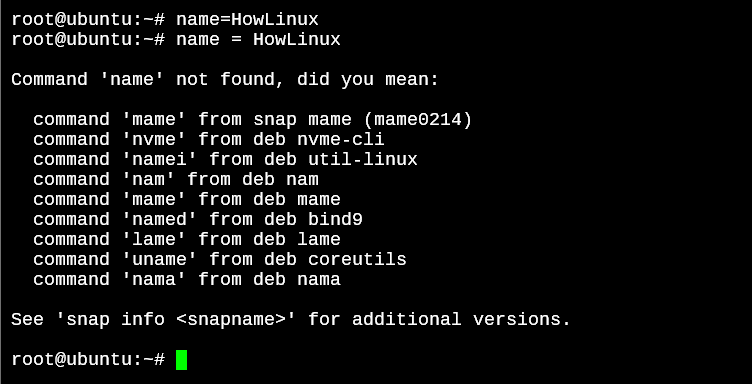
As you can see, the second time we got an error. So we know that variable assignments cannot have spaces in them. We already know from a lot of our previous tutorials, that Linux is a case sensitive operating system. So in the above case, the variable name is not the same as Name.
Printing Out Variable Values
Now that we have a value assigned to a variable name in the above example, it’s time to make use of it. We’ll print the variable with the use of the echo command. But how can we tell the echo command to print the value of the variable and not just whatever we type after it? We’ll use the $ symbol to indicate a variable when making use of it.
Let’s see what happens if we echo the variable name without the $ sign.
root@ubuntu:~# echo name
name
root@ubuntu:~# echo $name
HowLinux
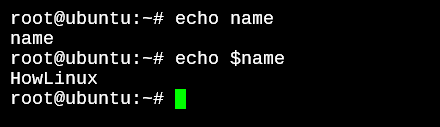
Reading User Input Into Variables
To read user input into a variable, we’ll use the read command followed by the name of the variable without the $ sign.
root@ubuntu:~# read name
HowLinux User Input
root@ubuntu:~# echo $name
HowLinux User Input
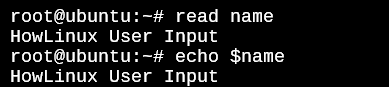
Saving and Executing Shell Script Files
Before we move on to accepting shell arguments, we’ll save the shell script in a shell file which can be then executed with the arguments passed to it.
The file extension for a shell script is .sh which very obviously, stands for “shell”. Let’s combine the script that we tested above in a file and run it.
#!/bin/bash
echo What is your name?
read name
echo Hello from HowLinux, $name.
Save this script above as input.sh. We’ll use the chmod command in Linux to make the shell script executable. This is optional because we can also execute the script by just typing bash input.sh
.
root@ubuntu:~# chmod +x input.sh
The script is now executable without the need of the bash command and can be executed by adding ./ at the beginning as shown below.
root@ubuntu:~# ./input.sh
What is your name?
Ninad
Hello from HowLinux, Ninad.
root@ubuntu:~#
Great! Now we know how to save and execute scripts. Let’s move into understanding some system variables, and how we can accept arguments passed to the script from the terminal.
Accepting Arguments in Shell Scripts
Now the thing is, even our input.sh script is already capable of accepting arguments. The question is not just about accepting, but about using the arguments passed to it. So let’s look into how we can use the arguments that are passed while executing a script.
Since in our input.sh, we won’t be able to demonstrate the usage of arguments, we’ll first create a new script, then come back to our input.sh and modify it to accept the name as an argument.
#/bin/bash
echo The script was called by using $0
echo First argument, $1
echo Second argument, $2
echo Third argument, $3
echo All arguments can be printed with $@
Save the above script as argument.sh and again run the chmod +x argument.sh
command to make the script executable.
root@ubuntu:~# ./argument.sh
The script was called by using ./argument.sh
First argument,
Second argument,
Third argument,
echo All arguments can be printed with
As you may notice, the $0 system variable tells us how the script was called. In this case, we simply called it by executing the script. If the entire path was specified, we’d get an output with the entire path. The $@ stores all the arguments together.
But we haven’t passed any arguments here. Let’s see what happens when we pass some arguments.
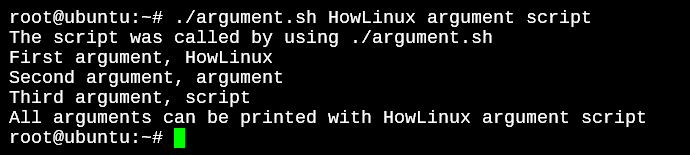
Exercise: Modify the input.sh script to accept the name as an argument instead of user input.
Special Variables in Shell Scripts
In the above write up, we saw a few special variables that can be used to access arguments that are passed to our scripts. Let’s see a few more special variables that can be used within our scripts.
- $$ – Gives you the process ID of the shell script when it’s run in the background. This is especially useful when you create a script that can run multiple times parallelly.
- $* – Similar to $@ in functionality as if stores and lets us output all the arguments passed.
- $# – Get the count of variables passed to the script
- IFS – This variable stands for Internal Field Separator and what it does is automatically uses SPACE, TAB, or NEWLINE characters as separators for inputs to a script. If you change this variable in the script to any other separator, your script will break down input using that character as a separator.
Conclusion
This is a shell script tutorial series and we’ll continue to write more articles on shell scripts in Linux. We hope you now understand how variables in shell scripts work and can make use of them too. Remember, anything that you can do on a terminal, can be done with a shell script, and more! So all the commands that you learned in our previous tutorials can be combined with the use of variables to automate some daily tasks. Let us know what you come up with!