Switch case in shell scripts is an efficient alternative to the if-elif-else statement we learned previously. The concept of the switch case statements is that we provide an expression for different cases (conditions) to the statement that, when fulfilled, will execute specific blocks of commands. Let’s learn how to create a switch case block and how it can be helpful to us in daily applications.
Basic Syntax of Switch Case in Shell Scripts
The switch case in shell scripts takes the variable as an argument and then checks the expression variable for a pattern match; a default case or condition will be used if no pattern match is found. Also, we can use multiple statements, but the minimum number of the statement has to be at least one. Have a look at the syntax below:
case expression/variable in
pattern1)
do-something-here
;;
pattern2)
do-something-here
;;
esac
Let’s say we want to check if a variable matches a name. Here’s what a sample of a multiple patterns based switch case statement would look like:
#!/bin/bash
name="HowLinux"
case $name in
"Linux")
echo "Not quite right"
;;
"HowLinux")
echo "That's right!"
;;
esac

As you can see, the string “That’s right!” is printed out because our variable matched that case statement. Here, the ;; command makes the program exit from the conditional script. Now let’s move on to some real-world applications of the switch case statement in bash.
A Real-World Application of Switch Case
When creating scripts for any purpose, we might also need to provide some script options that can be passed as arguments to our script. This is where our switch case can be used pretty easily. How do we do it? Let’s create a script of our own that accepts arguments and outputs values based on that.
What I’m going to do is to create a script that will allow us to create a file or delete a file based on the argument that we pass to it. But since we cannot handle everything that someone might pass as an argument, we will use the asterisk (*) operator to catch any other argument passed to it and print an error message.
#!/bin/bash
case $1 in
"--create")
echo "Creating new file $2"
echo
touch $2
;;
"--delete")
echo "Deleting file $2"
echo
rm $2
;;
*)
echo "Not a valid argument"
echo
;;
esac
The above script accepts an argument as –create or –delete, along with the name of a file. Based on the argument passed to the script when executing, the script will perform the action. Have a look at the screenshot below to see our short script in action.
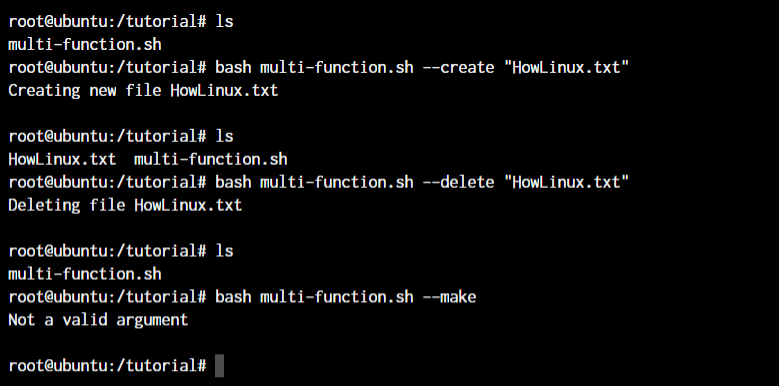
Conclusion
That’s it for our short tutorial on switch cases in shell scripts! With this article, we have learned how the switch case statements work in shell scripts which provides an easy way to correspond variables with different patterns and perform specific actions based on the matched pattern.
We will continue to add more and more functions as we move along, and I’m sure that by the end of this series, you’ll be a pro at shell scripting!
Hope this has been helpful to you. Feel free to add a comment below if you have any questions!