In our Shell scripting 101 today, we’ll learn how to make use of if else in shell script and what different situations require the use of the if and else statement in Linux. A demonstrated use of “if statement” was done in our previous tutorial about break and continue statements.
The different basic syntax for if else in shell script is:
- Basic if statement – if… [things to do if TRUE] …fi
- if-else statement – if…[things to do if TRUE]…else… [things to do if FALSE]…fi
- Multiple conditions – if….[things to do if TRUE]…elif…[secondary condition actions]…else…[things to do if FALSE]…fi
- Nested If – if… [things to do if TRUE]…else…if…[things to do if TRUE]…fi…fi
Basic If Statement in Linux
This is a simple conditional statement. When all you want your script to do is to check for a specific condition, you need a basic if statement. Let’s see how to make use of this.
n=1
if [ $n -eq 1 ]
then
echo "This is true"
fi
In the above script, all we’re doing is assigning the value 1 to the variable $n (learn more about variables in shell scripts), and then checking if the variable has the value of 1.
If it’s true, the statement “This is true” will be printed out. If it’s false, the script will output nothing because we do not have an else statement here.

You can also run the script by making it executable using the chmod command.
If Else in Shell Script
Let’s move on to a situation where the if condition becomes false. If we do not specify anything to handle this, the code will exit the “if” block and move on with the rest of the code. But we do not want that to happen. That’s where we make use of the else statement.
We’ll continue using the code above but we’ll change the value of $n.
n=2
if [ $n -eq 1 ]
then
echo "This is true"
else
echo "N is no longer 1"
fi
Save the above script and run it using the bash or sh command in your terminal.
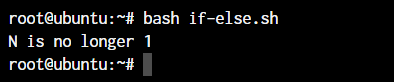
If we had not added the “else” block to the script, we would receive no output. The obvious reason is that we’ve not handled the situation for the script where $n is not equal to 1.
If Elif Else in Shell Script
Now we’ve handled one situation where $n is not equal to 1 using if else in shell script. But what if we have to handle a few more situations. Let’s say we need to identify if the variable is greater than 5 or not. If it’s greater than 5, then we ask the script to output something else. If it’s not equal to one, or not greater than 5, then we’ll resort to default output.
n=5
if [ $n -eq 1 ]
then
echo "Variable is 1"
elif [ $n -ge 5 ]
then
echo "The variable is greater than 5"
else
echo "Variable is greater than 1 but less than 5"
fi
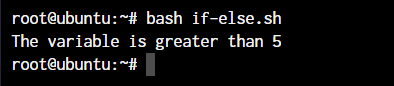
Try the above script and keep modifying the variables that are being used. You’ll see how the script flow runs from if to elif to else based on the variable value.
The if…elif…else…fi block can be used to handle a lot of different operations but a better option is switch…case which we’ll discuss in the next Shell Scripting 101 tutorial.
Testing Multiple Conditions in If Else in Shell Script using Boolean Operators
In the above code, we tested for one condition per statement. The if code tested if the $n variable was equal to 1. The elif (else if) condition tested if the variable was greater than or equal to 5, and the else statement handled the default output in case both the situations were false.
But we can also test for multiple conditions and not be limited to just one. Let’s say we want the if condition to test for $n=1 or $n=3, we’ll use the &&
(AND) or ||
(OR) boolean operators.
The && operator checks for both the operations to be true, while the || operator checks for either of the operations to be true. In our case, we’ll go with the || operation since the variable $n cannot be 1 and 3 at the same time.
n=3
if [ $n -eq 1 ] || [ $n -eq 3 ]
then
echo "Variable is 1 or 3"
elif [ $n -ge 5 ]
then
echo "The variable is greater than 5"
else
echo "Variable is greater than 1 but less than 5"
fi
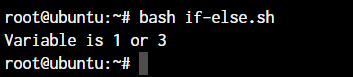
As you can see the if else in shell script checks for both the variable values and we’re able to handle two situations. We can do the same with the && boolean operator.
Conclusion
You made it! As we’re progressing through the tutorial, you should be able to create more and more complex scripts for yourself to automate a lot of your daily repetitive work. Make sure you continue to follow through the tutorials to understand shell scripting better.