In our previous tutorial, we learned the use of the while loop in shell scripts. Now, let’s make use of the break and continue statement in Linux and manipulate the flow of loops. As we know, a loop will end if the condition supplied to it becomes FALSE. But what if you’re running a loop that needs to stop or skip the rest of the loop based on certain conditions? This is where the break and continue statements in shell scripts are used.
What do the break and continue statements in Linux do? The break statement ends the flow of a loop as soon as the break statement is met. The continue statement, on the other hand, restarts the loop as soon as the continue statement is met with.
The break Statement in Linux Shell Scripts
We’ll first work on understanding how to break out of a loop that has more iterations left. I’ve created a while loop that should loop 50 times. But, I’ve added an if statement where as soon as the 25th loop is over, the break statement is executed.
a=0
while [ $a -le 50 ]
do
echo Loop $a
echo "Loop will break as soon as this is 25"
if (($a==25))
then
break
fi
((a++))
done
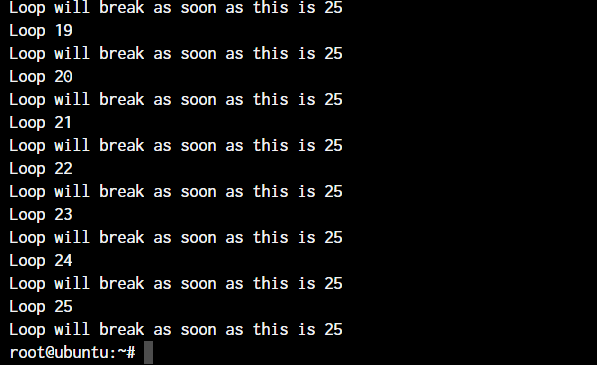
We’ll learn about if statements in the coming tutorials so for now, you can focus on getting used to the break statement. Notice how the loop stops right after the 25th loop.
As we mentioned before, a break statement will break the loop immediately. Anything after the break statement will not be executed. Let’s do a proof check of this here. I’ll add some echo statements here, after the if condition in the above script.
a=0
while [ $a -le 50 ]
do
echo Loop $a
echo "Loop will break as soon as this is 25"
if (($a==25))
then
echo "Breaking. Goodbye!"
break
fi
echo "Still not broken"
((a++))
done
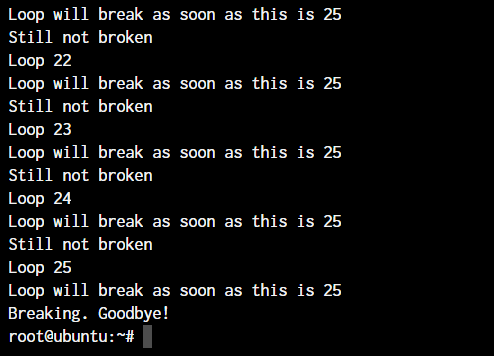
As you can see, even though we broke out of the loop on the 25th iteration, the “Still not broken” output is not printed on the screen since the break was placed before it.
In case you’ve nested loops, the break statement on its own will break only the loop that it was executed it. To break out of all the loops, we simply need to write the number of loops to break out of right after the break statement.
break N
So if you know the break statement is nested in 3 layers of loops for >> while >> while >> break, you can just say break 3 to break out of all the loops instead of just the last while loop where the break was executed.
This functionality is similar in both the break and continue statements in Linux.
The continue Statement in Linux Shell Scripts
The usage of the break and continue statements in Linux is exactly the same. The difference is in how they behave when executed. The continue statement, as we discussed before, will restart the loop ignore everything that’s after the continue statement within the loop.
This will let the loop finish the entire number of iterations that it is supposed to finish. All it will do is, for a certain condition, skip the entire loop after the continue statement to restart the loop. Let’s see it in action to understand it better.
a=0
while [ $a -le 50 ]
do
echo Loop $a
echo "Loop will break as soon as this is 25"
((a++))
if (($a==25))
then
echo "LET'S GO BACK TO THE START!"
continue
fi
echo "Still executing"
done
I moved the increment statement before the if statement. The reason is that if we had placed it right where it was before, the variable would never increment after 25 because the loop never reaches that part of the code.
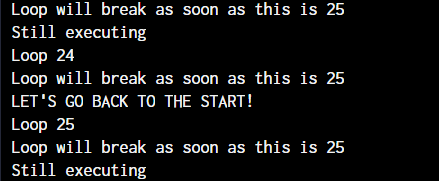
You see, on the 24th iteration, the if statement was triggered because the variable increments right before the loop.
If the continue statement is inside of a nested loop, we just need to pass an argument to the statement as we did for the break statement.
continue N
Here, N is the number of loops to break out of. So if there are 2 loops including the one the continue statement was executed it, we just say continue 2, and both the loops will restart.
Conclusion
This should be an easily digestible, short tutorial for you to get an understanding of how the break and continue statement in Linux works. If you need help in understanding variables, do make sure you read through our tutorial on variables and arrays in shell scripts. We’ll cover the if condition and for loops in our upcoming Shell scripting 101 series.