In the previous shell scripting 101s, we covered arrays and variables in shell scripts for Unix and Linux environments. In this tutorial, we’ll cover the while loop in shell script.
A while loop in shell scripts is used to repeat instructions multiple times until the condition for the loop stays true. Loops have a lot of use cases in real-world applications, since we create them to automate repetitive tasks. Let’s go over some of the use cases and learn to use the while loop in shell scripts.
Syntax of While Loop in Bash Scripts
The while loop works based on the condition supplied to it. It can be as simple as waiting for a variable to become equal to a specified number, or as complex as waiting for the output for another command to match the output we specified. The possibilities are endless here. If you can evaluate something to be TRUE or FALSE, you can use it as a condition for the while loop.
while [condition - should evaluate to TRUE or FALSE]
do
command
command
done
This is what a typical while loop looks like.
Boolean Operators When Working With Shell Scripts
Apart from the boolean operators that you’ve seen in other programming languages, including ==, <, >, ≤, >=, etc., these are shell script specific operators. The major usage difference is that these operators will only work when numeric values are provided. With string value, the operation will simply fail.
Operator | Description | Example |
---|---|---|
-ne | Checks if both operands are not equal | [ 1 -ne 2 ] is TRUE |
–eq | Checks if both the operands are equal | [ 1 -eq 2 ] is FALSE |
–lt | Check if the left operand is lesser than the right operand | [1-lt 2 ] is TRUE |
–gt | Check if the left operand is greater than the right operand | [1-gt 2 ] is FALSE |
–le | Check if the left operand is less than or equal to the right. | [1-le 2 ] is TRUE |
–ge | Check if the left operand is greater than or equal to the right. | [1-ge 2 ] is FALSE |
Creating a Simple While Loop in Shell Script on Linux
A while loop doesn’t have a repetition limit by default. We have to explicitly provide it a condition that, at some point during the code execution, will turn to false. Else you’ll have a loop that doesn’t end unless an interrupt signal is sent. Enter the following in a text file:
i=0
while [ $i -le 10 ]
do
echo True
((i++))
done
echo False
Save the script above by any name with the .sh extension. To run the file, you can either run it with the bash command like bash <filename>.sh or make the file executable using the chmod command. To make the script executable, run chmod +x <filename>.sh and then ./<filename>.sh will allow you to run the script.
Let’s understand the script line by line.
- i=0 – Here, we set the variable $i to 0. Learn more about variables in our previous tutorial
- while [ $i -le 10 ] – Run the while loop only until the variable $i is lesser than or equal to 10. So this loop will run 11 times, including the ZERO-th run.
- do – Marks the beginning of the while loop in shell scripts
- echo True – A simple command that will print the word “True” on our terminal
- ((i++)) – C style variable increments to add 1 to the variable i with every loop. There are various other ways to perform an increment include $i=((i+1)), ((i=i+1)), etc. Feel free to use either one.
- done – Marks the end of the while loop
- echo False – This again simply prints out the word False on the screen to indicate that the condition has now turned false.
You’ll receive an output similar to the one in the screenshot below.
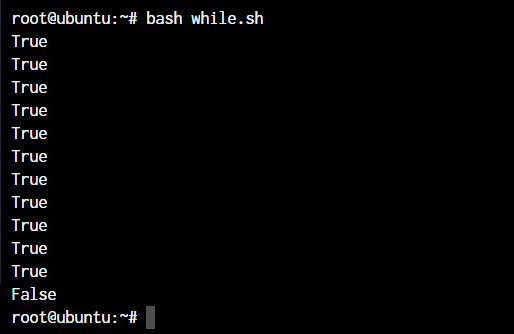
Infinite While Loop in Shell Script
Now that we figured how to add a condition to terminate the while loop, let’s see how we can work on an infinite while loop. The only thing we’ll have to do is to skip the condition part.
i=0
while :
do
echo Script ran $i times
((i++))
done
echo False
Save this script in another file with the .sh extension. You can follow the same steps as above to execute the shell script. As soon as the script is run, in less than 1 second, you should get the output 100s of thousands of times.
To stop the script, press Ctrl + C.
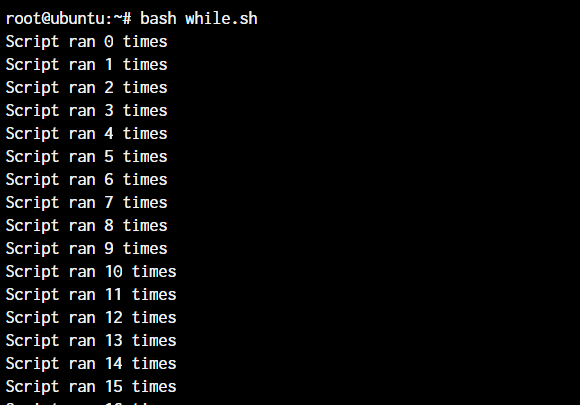
You’ll have to scroll way up to even see where you started because the loops run very quickly.
Conclusion
This is a simple enough loop to not need detailed coverage at present. As we move ahead with topics, we’ll continue to use the while loop on a consistent basis for more detailed operations including reading files, evaluating more conditions for the user. When you follow through with the shell scripting 101 tutorials, you will learn one topic with every tutorial.
Make sure you practice enough to make sure you’re well versed and have a good hands-on before moving to the next topic. In this tutorial, we’ve covered the while loop in shell script and the usage.
References
https://www.gnu.org/software/gawk/manual/html_node/While-Statement.htm