In the previous shell scripting tutorial, we worked with simple variables in shell scripts. Today we’ll cover arrays in shell scripts, and how we can create and make use of arrays within our scripts.
To declare or create arrays on the fly, we will use the declare command (more explicit) or directly write array_name=(element1 element2 ...)
. There are other ways we can create and access arrays and in this tutorial, we’ll go into as much detail as possible.
What are Arrays in Shell Scripts?
Variables can store single values. Arrays, on the other hand, can store virtually unlimited values in it where each value is indexed starting from index 0 for the first value.
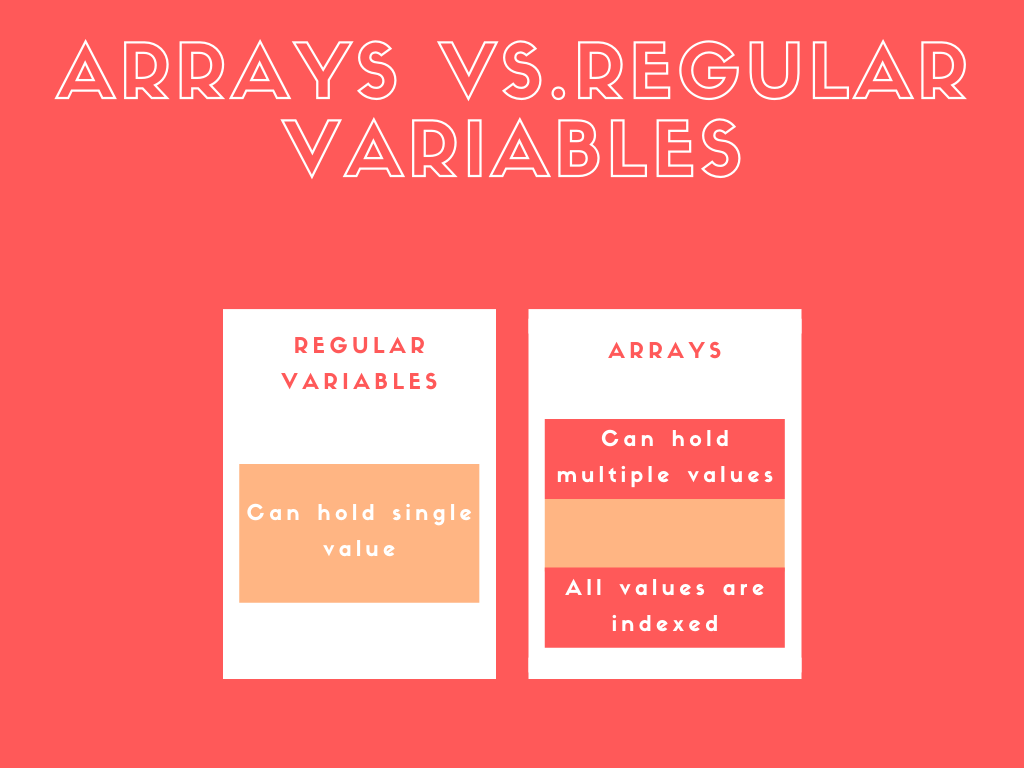
When you’re creating scripts that interact with a lot of values, it’s more efficient to use arrays instead of creating a lot of individual variables.
How to Create Arrays in Shell Scripts?
We can either declare an associative or indexed array and then assign the values, or directly create an array with the values. Let’s see an example of all three cases.
Arrays are of the format array_name[subscript]=value.
To create an indexed array, we’ll use the declare command with the -a option. An indexed option does not require you to explicitly declare a subscript. If you specify a subscript, the array uses it. When no subscript is specified, it will automatically index the elements starting with 0.
root@ubuntu:~# declare -a index_array
root@ubuntu:~# index_array+=("first item")

For an associative array, we will use the -A option of the declare command. With associative arrays, we need to explicitly specify what the subscript will be else we receive an error.
root@ubuntu:~# declare -A assoc_array
root@ubuntu:~# assoc_array+=("first item")
-bash: assoc_array: "first element": must use subscript when assigning associative array
root@ubuntu:~# assoc_array+=([first]="first item")

As you can see, the associative array simply won’t accept the values unless they are provided in a key-value pair. But you do not need to declare an array beforehand. You can simply assign the values to the array.
Now let’s work with an array that we assign values to on the fly without declaring the name first.
root@ubuntu:~# array=("first item" "second item")
root@ubuntu:~# array2=([first]="first item" [second]="second item")

Both of the commands above are correct and the arrays in shell scripts will automatically be “typed” to associative or indexed arrays based on the values provided.
Outputting Array Values
Now that we’ve learned how to create arrays in shell scripts, let’s see how we can output them. We’ll continue to use the echo command to see different ways of outputting an array.
Let’s use the echo command on the array variable that we built on the fly: array. The $ notation is used to signify an array (refer to the article on variables in a shell script to learn more)
root@ubuntu:~# echo $array
first item
As you can see, it only outputs the first item. But why is that so? If this was a variable, we would have received the entire output.
The reason why it only outputs the first item is that when we do not specify an index when trying to output arrays in shell scripts, it will assume an index [0]. So we will specify [@] or [*].
root@ubuntu:~# echo ${array[@]}
first item second item
Now you might notice the use of curly brackets here. These are being used to explicitly mention that the entire part starting from array to [@] is all to be considered as one. If we do not use the curly brackets, here’s what the output will be.
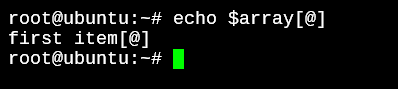
Makes sense? Great! Now let’s move on further.
How to print a range of elements? We can do this without much effort by using the index section to slice the output.
${array_name[ELEMENTS-USUALLY JUST @ or *]:START-INDEX:NUMBER-OF-ELEMENTS}
root@ubuntu:~# echo ${array[@]:0:1}
first item
If we remove the NUMBER-OF-ELEMENTS, the output will print all elements starting from the START-INDEX.
Various Array Operations in Shell Script
We can count, delete, and replace items within the array with real easy commands. Let’s see how.
How to Count the Number of Elements in Arrays
There are times when we might need to know the exact number of elements for arrays in shell scripts where the elements are added on the fly. I’ll add a hash/pound sign (#) to the echo command that we used before to print the entire array. This will give us the number of elements in an array.
root@ubuntu:~# echo ${#array[@]}
2
As you already know, we have two elements in our array.
How to Count the Number of Characters of an Array Element
Similar to the example above, if we change the [@] with the index of the element, we’ll get an output as the number of characters.
root@ubuntu:~# echo ${#array[1]}
11
Replace Specific Characters From an Array
When using arrays in shell scripts, there will be times when we need to make changes to existing elements in the array on the fly. Similar to find and replace, we can pattern match and replace specific characters or words in an array or an array element. Let’s replace the word “item” with “ITEM” in our array.
root@ubuntu:~# echo ${array[@]}
first item second item
root@ubuntu:~# echo ${array[@]//item/ITEM}
first ITEM second ITEM

Delete an Array Element or Entire Array
When dealing with multiple arrays in shell scripts with various elements, there are times when you’d need to make sure that values or entire arrays are eliminated from the script. To delete an element from an array, we’ll use the unset command and specify the index of the element.
root@ubuntu:~# echo ${array[@]}
first item second item
root@ubuntu:~# unset array[1]
root@ubuntu:~# echo ${array[@]}
first item
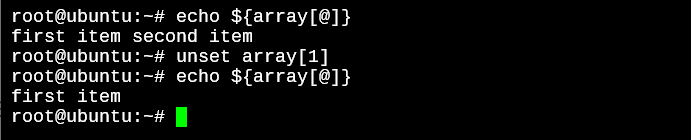
If you’d like to delete entire arrays in shell scripts, you can use the unset command without an index.
root@ubuntu:~# echo ${array[@]}
first item
root@ubuntu:~# unset array
root@ubuntu:~# echo ${array[@]}
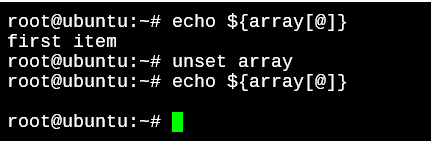
What is the purpose of using arrays in shell scripting?
Arrays in shell scripting allow you to store and access multiple values under a single variable. This can be incredibly useful for managing and manipulating large sets of data.
How do I declare an array in a shell script?
You can declare an array in a shell script using the following syntax: declare -a my_array. This creates a simple indexed array named my_array.
Can I use arrays in bash shell scripting?
Yes, arrays are fully supported in bash shell scripting. You can declare and manipulate arrays using the standard bash syntax.
How do I add elements to an indexed array in a shell script?
To add elements to an indexed array, you can use the syntax my_array+=(“element”). This will append the new element to the end of the array.
What is the difference between an indexed array and an associative array?
An indexed array uses numeric indexes to access its elements, while an associative array uses keys and values. Indexed arrays are accessed using numbers, while associative arrays are accessed using specific keys.
How can I print all the elements of an array in a shell script?
You can print all the elements of an array in a shell script using the syntax echo ${my_array[@]}. This will display all the elements contained in the array.
Can I create a simple array consisting of string elements in a shell script?
Yes, you can create a simple array containing string elements in a shell script. Simply declare the array and add the elements using the appropriate syntax.
How do I determine the length of an array in a shell script?
To find the length of an array in a shell script, you can use the syntax ${#my_array[@]}. This will return the number of elements in the array.
What is the process for creating an associative array by specifying keys and values?
To create an associative array with keys and values, you can use the syntax declare -A my_array. This sets up my_array as an associative array, and you can then add elements using specific keys.
Conclusion
This tutorial should help you get up and running with arrays in shell scripts. In future tutorials, we’ll cover loops and conditions which will allow you to interact with arrays in a much better manner.
References for this article:
https://www.gnu.org/software/bash/manual/html_node/Arrays.html