Python is a trendy & modern programming language. Especially popular among beginners as it is easy to learn. Python is so powerful that we can use it to interact with the operating system. In Linux, python act as an alternative for bash command language for scripting. It comes preinstalled in most of the distributions as it is a dependency on many tools and software. And if not it is effortless to install.
In this tutorial, we will see how to run Linux commands using Python. The idea behind this is to automate tasks and save time. I am using Ubuntu for this tutorial.
Also read: Installing Latest Python on Debian/Ubuntu using Source
Prerequisites
We will require a python environment. If you are using the latest distribution it is preinstalled. You can check using the following command.
❯ python3 --version
To install python run the following command –
❯ sudo apt install python3 -y
Additionally, if you have some knowledge of terminal & some Linux commands that will be great.
The OS module in Python 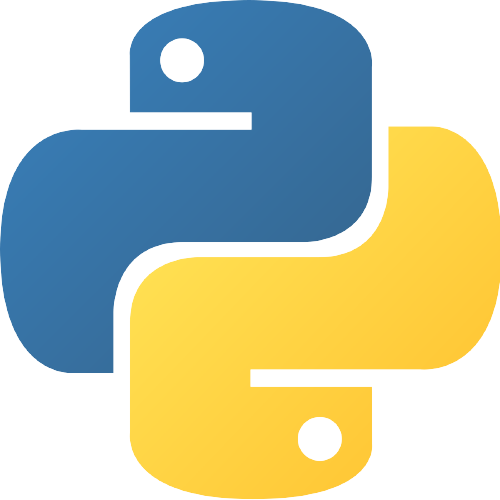
OS library provides operating system-based functions and allows us to interact with the operating system. The package comes with python installation, so no need to install it separately. The library is easy to use and contains both read and write operations.
Let’s write our first program and get system information –
Launch your favorite text editor (I am using gedit) –
gedit system_info.py
Copy the below code and save the file –
import os
os.uname()
You can run the file like the below –
python3 system_info.py
Output
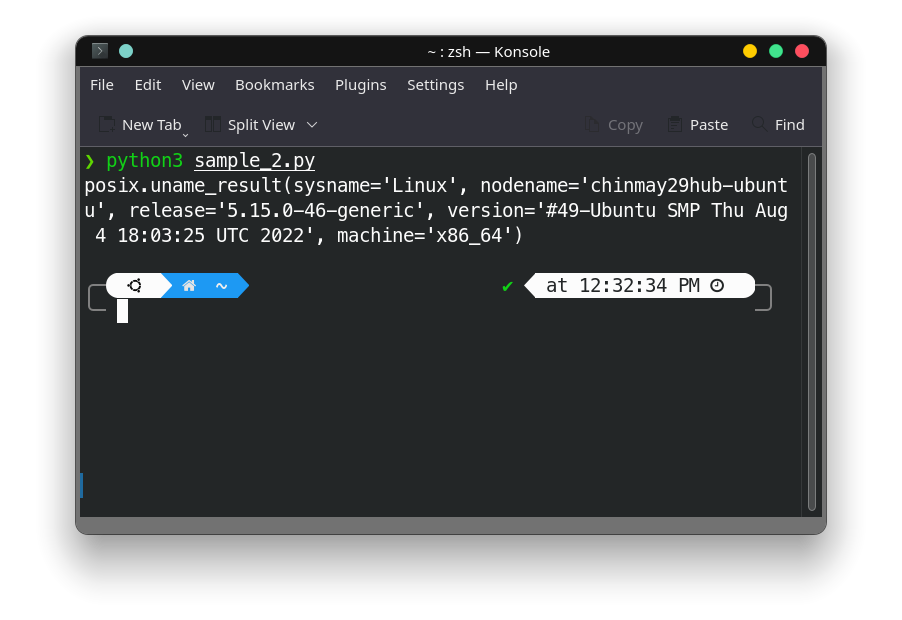
The uname()
method returns information like name, release, and version of the current operating system, etc. This was just an overview of the OS module, now let’s run the terminal commands.
os.system()
The os.system()
method takes command as a string and executes it in a subshell. The limitations stand the same as the system()
method in C language. The method is system dependent.
The code should be executed using the terminal as if you run the code in IDLE you get “0” as output and nothing really happens.
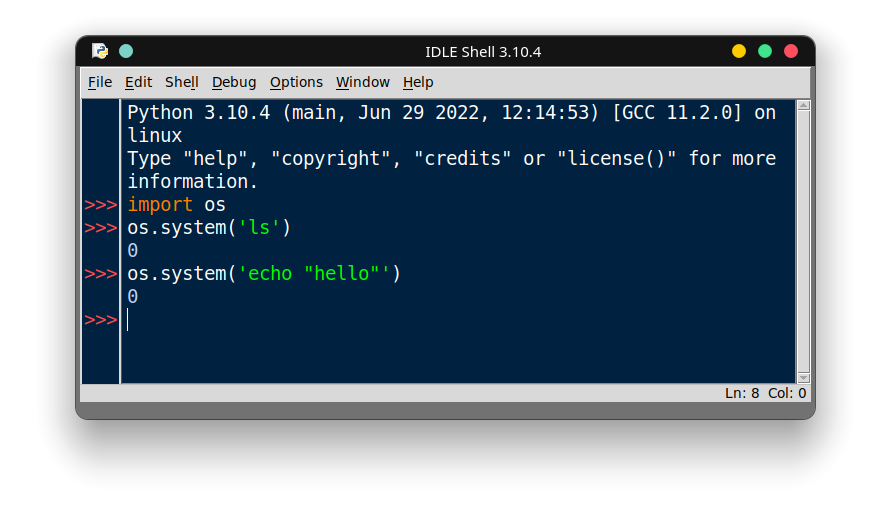
Let’s run our very first command –
import os
os.system('ls')
Output
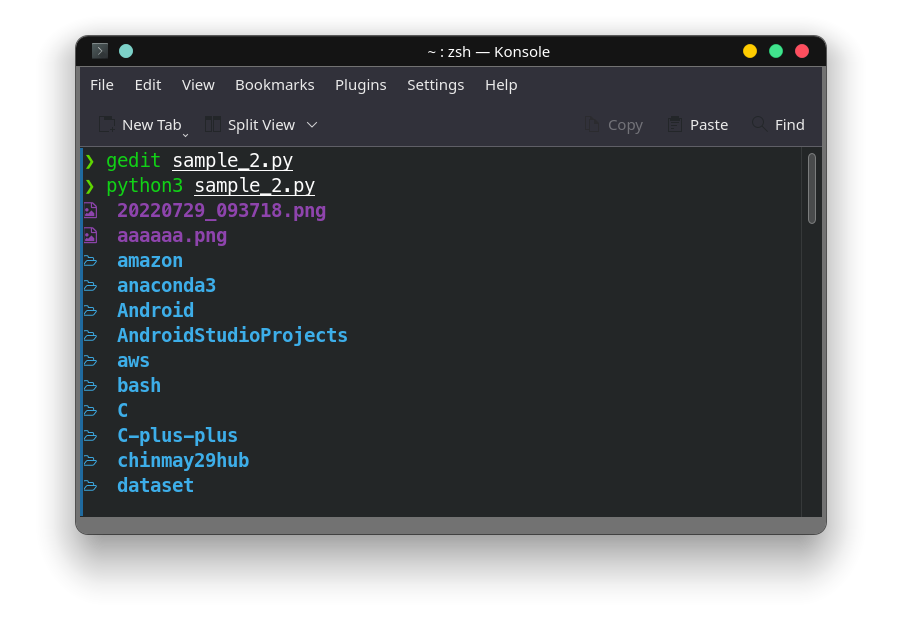
The ls command is used to list items in the respective directory.
Accept Linux Command As User Input
Let’s take input from users and then concatenate it with our command. For eg: Creating folders while taking folder names from the user.
Code
import os
name = input("Enter a folder name : ")
print("\n")
os.system('mkdir ' + name)
Output
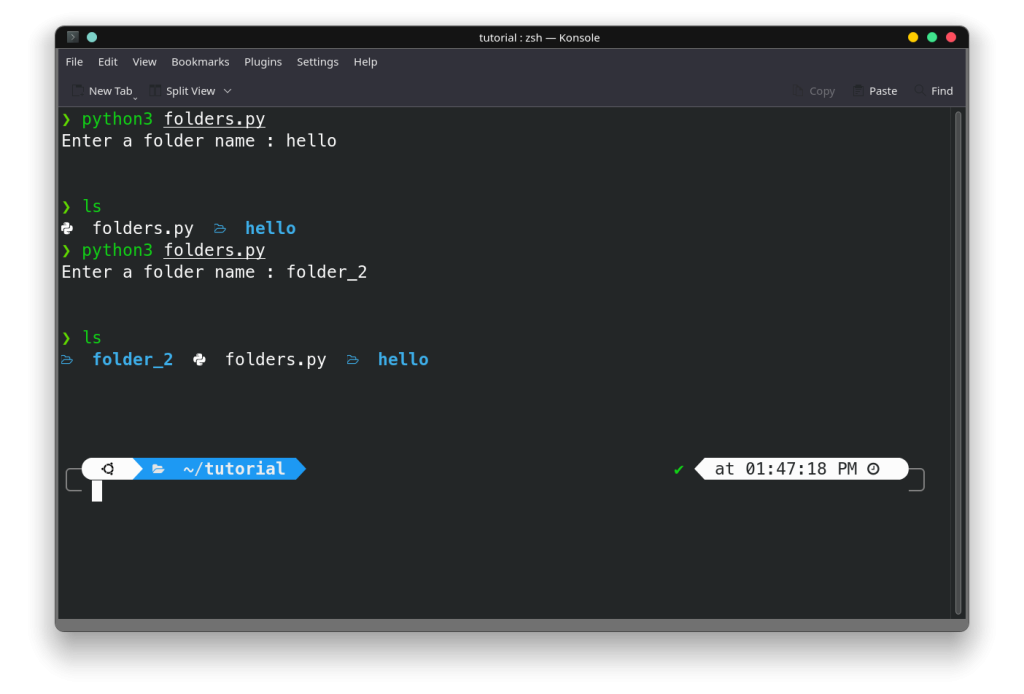
Complete Code for Executing Linux Commands Using Python
I have developed a simple script to give you guys an idea of how you can use python to make your job easy.
import os
while (True):
print("1) List Directories")
print("2) Create a file")
print("3) Create a directory/folder")
print("4) Ping a server")
print("5) Neofetch")
print("6) Check Python Version")
print("7) Check Java Version")
print("8) Exit")
ch = int(input("Enter Your Choice : "))
if ch == 1:
os.system('lsd')
elif ch == 2:
f_name = input("Enter file name : ")
e_name = input("Enter file extension : ")
os.system('touch ' + f_name + '.' + e_name)
elif ch == 3:
name = input("Enter folder name : ")
os.system('mkdir ' + name)
elif ch == 4:
s = input("Enter address(Press enter for default - google.com) : ")
p = input("Enter number of packer(press enter for default - 10 : )")
o = input("Do you want the output in a txt file -- y | n -- :")
# p = int(p)
if (s == ''):
s = 'google.com'
if (p == ''):
p = '10'
if (o == 'y'):
os.system('ping ' + s + ' -c ' + p + ' > ping-output.txt')
else:
os.system('ping ' + s + ' -c ' + p)
elif ch == 5:
os.system('neofetch')
elif ch == 6:
os.system('python --version')
elif ch == 7:
os.system('java --version')
elif ch == 8:
break
else:
print("Invalid Choice")
This will show a menu to the user and the user has to choose any one option. As the script is in a while loop the menu will be presented to the user continuously. You can add more options to the menu.
Output
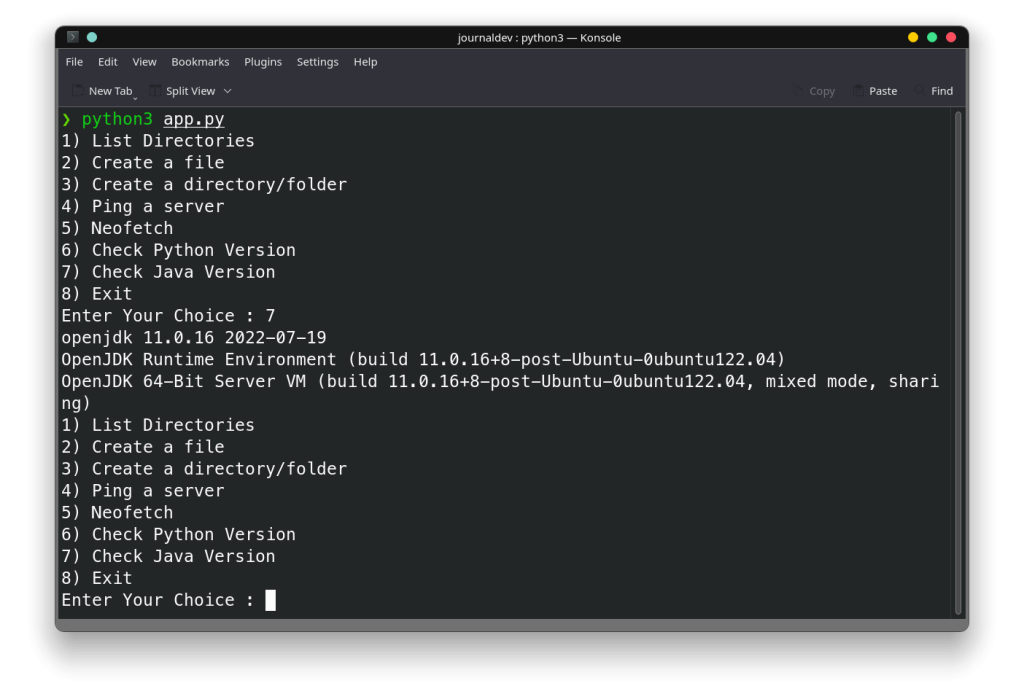
Wrapping Up!
This was just a small part of the OS module. It has many more features and methods. You can set the path of the scripts you have developed in the shell configuration file. This will allow you to run the scripts from anywhere. Also, create cron jobs to execute automatically from time to time. Hope this article helped you in some way.
Articles You Might Be Interested In
- Coding A Reverse Shell In Python In 10 Lines Or Less
- How to Configure Automatic Updates using Cronjob?
- How To Run Commands in Linux Every X Seconds?
Linux Command
A Linux command is a specific instruction or action given to a computer operating on a Linux system. It is a fundamental component for interacting with the operating system through a command-line interface.
Executing Commands in Python
Executing commands in Python allows you to run Linux commands within a Python script. This can be achieved using the subprocess module to execute commands in the operating system’s shell.
Output in Python
Output in Python refers to the result or response generated by the execution of a command or code snippet. You can capture and display this output using Python’s subprocess module.
Using the Subprocess Module
Using the subprocess module in Python allows you to create new processes, connect to their input/output/error pipes, and obtain their return codes. It provides an interface for executing system commands or scripts from within a Python program.
Error Handling
Error handling in Python involves managing and addressing any issues or exceptions that occur during the execution of code or commands. You can use try-except blocks to capture and handle errors effectively.
Capturing Command Output
Capturing command output in Python involves storing the result of executing a system command. This can be achieved using the subprocess module to run commands and capture their output for further processing.
Executing Commands Safely
When executing commands in Python, it is essential to do so safely to prevent any security risks or unintended consequences. Always validate user input and use secure methods to execute system commands.
What should I keep in mind when executing shell commands in Python?
When executing shell commands in Python, it’s important to properly handle the stdout and stderr outputs, ensuring that your script behaves as expected.
Who can benefit from learning how to execute Linux commands in Python?
Anyone from developers to system administrators can benefit from learning how to execute Linux commands in Python, as it opens up a wide variety of possibilities for automating tasks and managing systems.