We talked about the while, for, and until loops in shell scripts in the past few tutorials now. Let’s learn the select loop in shell scripts, a very interesting and unique loop in bash. Why is it interesting or unique? Let’s find out in this tutorial today.
What is the select loop in Shell Scripts?
The select loop is an infinite loop that only ends when there’s a keyboard interrupt or a break statement is encountered. But that’s not what makes it unique or interesting. The select statement allows users to choose from multiple options by default and it will prompt the user for an input. You do not have to write any code to accept user input as the select loop is pre-built to handle it.
This loop can be used to make menus within your script while keeping the script looping infinitely. Another benefit of the select loop in shell scripts is that it can be combined with the switch case statements to create really interactive menus or script pivots. Let’s learn how to make use of this loop and work with it.
Creating a select loop
The syntax for creating a select loop is very similar to the for loop
when looping over a list of items. Have a look at a basic select loop below.
select <variable> in <list of items separated by space>
do
... What to do for each option or with the variable ...
done
Let’s create a real select loop and see an example output:
#!/bin/bash
PS3="Enter your choice ==> "
echo "What do you do?"
select answer in Student Businessman Professional Fresher
do
echo "Great! You're a $answer!"
done
The PS3 variable
decides the prompt which will be displayed when the select loop waits for user input.
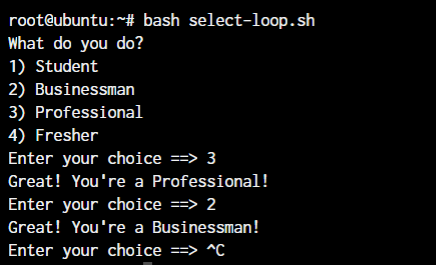
Isn’t this really amazing? The loop organized and created a really good looking options menu for us without us having to do anything!
Handling Inputs Individually in the select Loop
As you saw in the above example, the output was the same for all the 4 options that we had. Let’s understand how we can handle the inputs separately. For this, we’ll make use of the case statement in Linux. We can also use the if-elif-else clause but as we’ve seen in our previous tutorials, the switch case statements make more sense in multiple option scenarios.
#!/bin/bash
PS3="Enter your choice ==> "
echo "What do you do?"
select answer in Student Businessman Professional Fresher
do
case $answer in
Student)
echo "You're still studying"
;;
Fresher)
echo "You're new in the job market"
;;
Professional)
echo "You've been a seasoned professional"
;;
Businessman)
echo "Wow you work really hard for sure!"
;;
*)
echo "Well, you need to enter something from the list!"
;;
esac
done
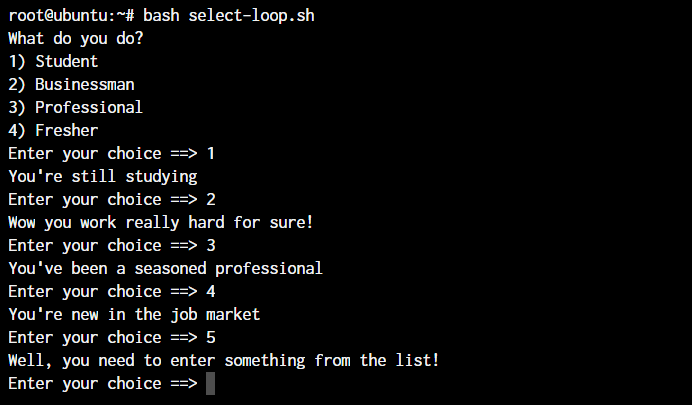
Modifying the Input Prompt in Bash
This is not just related to the select loop in shell scripts. The PS3 variable is an environment variable that stores what your bash prompt should look like. You can export this variable to have any other information that you wish to display on your bash prompt or just to make it look better.
But in relation to select loops, you can simply initialize the PS3 variable to have the prompt that you want the script to show. In my case, I’ve used PS3="Enter your choice ==> "
which is exactly what the select loop prompt is displaying.
Conclusion
I hope this tutorial about the select loop has been useful to you. We’ve now covered all the loops that are available in shell scripts. Don’t hesitate to comment below if you have any questions in regards to the select loop.