Moving ahead with our tutorials now, let’s introduce the for loop in shell scripts in our today’s topic. We’ve already covered the while loop in our previous tutorial and used the break and continue statements to quit the while loop based on certain conditions. Let’s get right into the topic then!
What’s The Difference Between while Loop and for Loop in Shell Scripts?
The major difference between for loop and while loop is in their definition and syntax. When working with the while loop, we provide one condition that the while loop continues to loop on until it turns false. We do not specify the number of iterations for the loop. On the other hand, for loop takes 2 or 3 conditions and the last condition usually decides the number of iterations.
The first condition is the variable that iterates through a sequence when the loop begins and increments automatically based on what it’s iterating over. For example, if it has to run over a sequence of numbers, the variable increments itself based on the numbers supplied to it.
If everything I wrote above sounded like gibberish to you, just continue reading and everything will become crystal clear soon.
Definition of the Bash for loop
There are two ways you can define the bash for loop. The first is the bash style with two variables, and the second is C style with 3 variables. Here’s the syntax for both of them.
Bash Style for loop definition:
for VARIABLE in SEQUENCE
The VARIABLE is the variable name to use within the loop, and the SEQUENCE is anything ranging from a sequence of numbers, multiple files, Linux commands, etc.
C Style for loop definition:
for (( variable declaration; condition; increment variable))
How to Use the for Loop?
Now that you have a basic understanding of the for loop, it’s time to start using it in our scripts. We’ll start by creating a simple for loop that iterates over a given sequence of numbers.
#!/bin/bash
for i in 1 2 3 4 5 6 7
do
echo "Loop number $i"
done
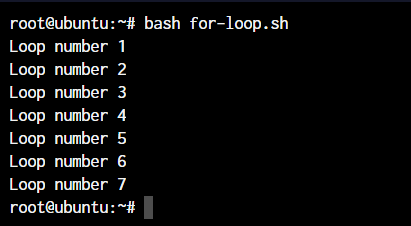
Very simple to understand? Let’s move on to a different sequence group.
Bash For Loop Over Files
The for loop doesn’t read the contents of the file when we loop over it but just allows us to perform operations on individual files while looping over them. Let’s see an example below.
#!/bin/bash
for i in *.txt
do
echo "Contents from the files: $i"
cat $i
done
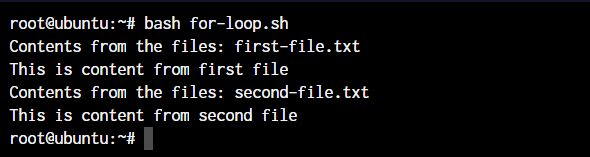
I’ve created two files with some text in them for demonstration purposes. See how I write the cat command once and the for loop iterates over both the files.
Writing C Style for Loops in Shell Scripts
You should be comfortable with iterating over individual sequences now. Let’s move on to the C style for loops that we talked about before. If you’ve already worked with C or C++, this is going to be almost the same thing. But even if you haven’t it’s not too difficult.
Let’s create a for loop that iterates 7 times and echos the number.
for (( i=1; i<=7; i++ ))
do
echo "Loop number $i"
done
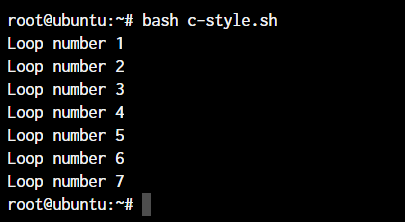
Let’s understand the loop section by section. The reason why we use the double parenthesis is to signal bash that we’re using a C style expression within the brackets.
- The first expression: i=1 creates the start of the loop.
- The second expression: i<=7 tells that the loop should run until the variable is lesser than or equal to 7
- The last expression: i++ decides how the increment happens. In this case, it happens by 1
Conclusion
I hope this helps you understand how the for loop in shell scripts works. Feel free to comment below for any questions that you may have.