When you type a command in the Linux Terminal, you expect an output. But sometimes you might encounter ‘Bash: Command Not Found’ error in the terminal when you run a command. This usually occurs when Bash cannot locate the file you’re attempting to execute in the specified location indicated by the path environment variable. Let’s explore what an environment variable is and how to change the variable to ensure we don’t face the command not found error on any Linux distribution such as Ubuntu or Fedora.
What is an environment variable?
An environment variable is like a little note or reminder that your Linux system uses to remember important information. Imagine you have a bulletin board where you pin notes to remember things like your favorite color, where you saved a file, or your best friend’s phone number. Similarly, your computer uses environment variables to store and remember certain details that various programs, binary files, scripts, or applications can access when needed.
These details could be settings, paths to specific files, or other important data. By using environment variables, it becomes easier for programs to communicate with each other and share important information without you having to remember all the details yourself.
There are mainly two types of environment variables: system-level and user-level environment variables.
Each serves as a kind of memo for your computer but differs in scope and accessibility.
System-level environment variable
System-level environment variables apply to the whole system and are accessible by all users and applications on the computer. They typically store crucial information for the system to function properly, like the location of system files or default settings for certain programs. Administrators can change these variables, but regular users usually can’t. Some examples of system-level variables include:
- PATH: This path variable directs the system to the folders where it should search for executable files, also called as the system’s PATH.
- SystemRoot: This variable points to the folder where the operating system is installed.
- TEMP and TMP: These variables indicate the folders storing temporary files.
User-level environment variable
User-level environment variables, on the other hand, are specific to each user account on the computer. They store information relevant only to that particular Linux user and their applications. Users can modify their user-level variables without impacting other users or the overall system. A few examples of user-level variables are:
- HOMEPATH: This variable points to the user’s home directory, where their files and settings can be found.
- USERPROFILE: This variable specifies the location of the user’s profile folder, containing their settings and data.
- APPDATA: This variable directs to the folder where applications store user-specific data and settings.
Use of the ‘export’ command
In bash, system-level variables or environment variables don’t persist without the export command because when you set a variable without export, you create a local shell variable instead of an environment variable.
Local shell variables are only visible within the shell process where they are defined. They are not passed on to child processes, or other applications started from that shell.
When you use the export
command, you are explicitly telling the shell to make the variable an environment variable, making it available to other processes, including child processes and programs launched from the shell. The export
command marks the variable for export to the environment of any subsequently executed commands.
If you want to persist a system-level variable across sessions or system reboots, you need to set and export the variable in a shell startup file, such as ~/.bashrc
, ~/.bash_profile
, or /etc/profile
(depending on your use case and the specific shell).
What is the PATH environment variable?
The PATH environment variable in bash is a special variable containing a list of directories separated by colons. When you try to execute a command without specifying its full path, the shell searches these directories for the executable file corresponding to that command.
The PATH variable allows users to run programs or scripts without providing the full path to the executable file. When you enter a command, the shell checks each directory listed in the PATH variable in the order they appear, looking for a matching executable. Once it finds the executable, it runs the command.
To view the current PATH variable in your bash shell, you can use the echo
command:
echo $PATH
This will output the list of directories currently included in the PATH variable. An example PATH variable might look like this:
/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
In this example, the shell will search for executables in the following directories in order:
- /usr/local/sbin
- /usr/local/bin
- /usr/sbin
- /usr/bin
- /sbin
- /bin
To add a new directory to the PATH variable, use the following syntax:
export PATH="$PATH:/new/directory/path"
This will append the new directory path to the existing PATH variable without overwriting its current value.
In the tutorial, we will examine why the command not found error occurs and how we can resolve this issue.
Fixing the Command Not Found Error in Linux
This error means that the command you entered is not recognized by the shell (bash) because either that command is not installed or you are typing a command that does not exist. This could also be due to misspelled command. Let’s check how we can fix this error.
Method 1. Check The Spelling
You might have made a spelling mistake while typing the commands. Even I sometimes become confused between I (Capital i) and l (Small L) and 1 (numeric 1). Ensure that you have entered the correct command name and used the spaces and options in the command correctly. For example, here, I have typed the wrong command, and therefore I have encountered the error.
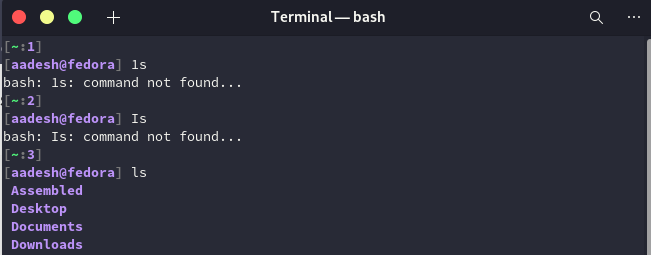
Method 2. When the Command is not installed
Maybe the command you are trying to use is not installed on your system. In that case, install the command using your appropriate package managers, such as apt, Pacman, DNF, or zypper. For example, I don’t have nano installed on my system because I use the Vim text editor. But if I try to open my .bashrc file using nano, It will show me the bash error that it’s not available. I can then install nano using the following command:
sudo apt install nano #For Debian based distributions
sudo dnf install nano #For Red hat based distributions
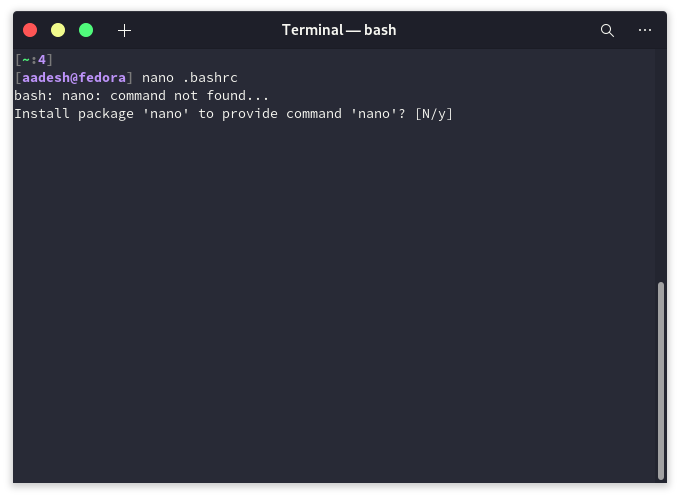
Method 3. When the command in use is an Alias
We often store our commands in the bashrc file as an alias so we do not have to type long commands often in the command line. But this can create problems for you, especially when you log in to the same computer using another user ID or accessing any other computer. In that case, try to find out the correct command for what you’re trying to achieve. You can also go back to your PC/User and type the following command to know all the aliases stored for you in the bashrc file:
alias

Now you can copy-paste the actual commands in the Terminal.
Method 4: Incorporate the File Path You Wish to Execute
Suppose the command you want to run is a script or executable file not located in one of the directories listed in your PATH environment variable. In that case, you can specify the entire path to the file when running the command. This way, the shell knows exactly where to find the file, and you won’t get the ‘command not found’ error message.
For example, if you have a script called “myscript.sh” in the /home/username/scripts directory, you can run it by providing the full path:
/home/username/scripts/myscript.sh
Method 5: Incorporate an Additional Path
You can add a directory to your PATH environment variable if you have a directory containing several executable files or scripts you want to run frequently. This will make the shell aware of the files in that directory when searching for commands to run.
To add a new path, you can edit your bashrc or bash_profile file in your home directory and add the following line:
export PATH=$PATH:/path/to/your/directory
Replace “/path/to/your/directory” with the actual path to the directory containing the commands.
Method 6: Transfer a File to a Pre-existing Path Location
If you have a single executable file or script that you try to run frequently, you can copy it to one of the directories listed in your PATH environment variable. This way, the shell can find and run the command without specifying its full path.
For example, if you have a script called “myscript.sh” and you want to copy it to /usr/local/bin, which is a common directory for custom scripts, you can use the following command:
sudo cp /path/to/myscript.sh /usr/local/bin
Make sure to replace “/path/to/myscript.sh” with the actual path to the script.
Method 7: Direct Bash to the Desired Location
Suppose you know the command you want to run is located in a specific directory, but you don’t want to add it to your PATH permanently. In that case, you can use the ‘source’ or ‘.’ command to include the directory in the shell’s search path temporarily.
For example, if you have a bash script called “myscript.sh” in the /home/username/scripts directory, you can tell Bash to look in that directory by running:
source /home/username/scripts/myscript.sh
or
. /home/username/scripts/myscript.sh
This will execute the script and inform the shell of any functions or aliases defined for the current session. Note that this change will not persist across different terminal sessions, so you must run the ‘source’ or ‘.’ command again if you open a new terminal or restart your computer.
Summary
There certainly isn’t one way to fix this problem, as there could be several reasons causing this problem in the first place. Hopefully, the above-mentioned methods were able to fix this error for you.
What is the typical reason for encountering a “Command Not Found” error in Linux?
The most common reason for this error is when the system cannot locate the command you are trying to execute.
How can I troubleshoot a “Command Not Found” error in Linux?
You can troubleshoot this error by checking your PATH variable, ensuring correct permissions, or by locating and adding the command’s location manually.
Why am I unable to find the command I need in my Linux shell session?
If you can’t find the command you’re looking for, it might not be installed on your system or its location may not be included in your PATH variable.
Can a typo in the command result in a “Command Not Found” error?
Yes, a simple typo or case sensitivity issue can lead to the system not being able to locate the command properly.
What should I do if I encounter a “Command Not Found” error for a commonly used command like ‘ls’ or ‘update’?
In the case of widely used commands, you should first ensure they are installed on your system. If they are, try adding their location to the PATH variable.