In this article, we’ll build and execute C++ programs using the terminal. Are you a terminal lover who’s fond of working with C/C++ programs? Prefer nerdy over the mainstream? If yes, then sit tight I’ve got something very interesting for you guys.
Also read: 4 Best Terminal Based Text Editors for Linux
Quick Summary
In this article, we’ll learn how to use the Terminal to build and execute C/C++ programs with ease. We’ll also learn the meaning and importance of each flag passed to the compiler while compiling our program.
Understanding Flags
Before we jump into the topic, let’s go over the concept of flags. If you are aware of this part, you can skip to the next section.
Flags are additional arguments passed to some program while issuing a command in the Terminal. There can be a large number of flags defined for a particular program. Each of these flags serves a specific purpose. This will become more clear to you after the following example:
The best way to search for flags that can be passed to a program/command is by using the “--help
” flag. This flag is defined for almost all the terminal commands.
g++ compiler flags
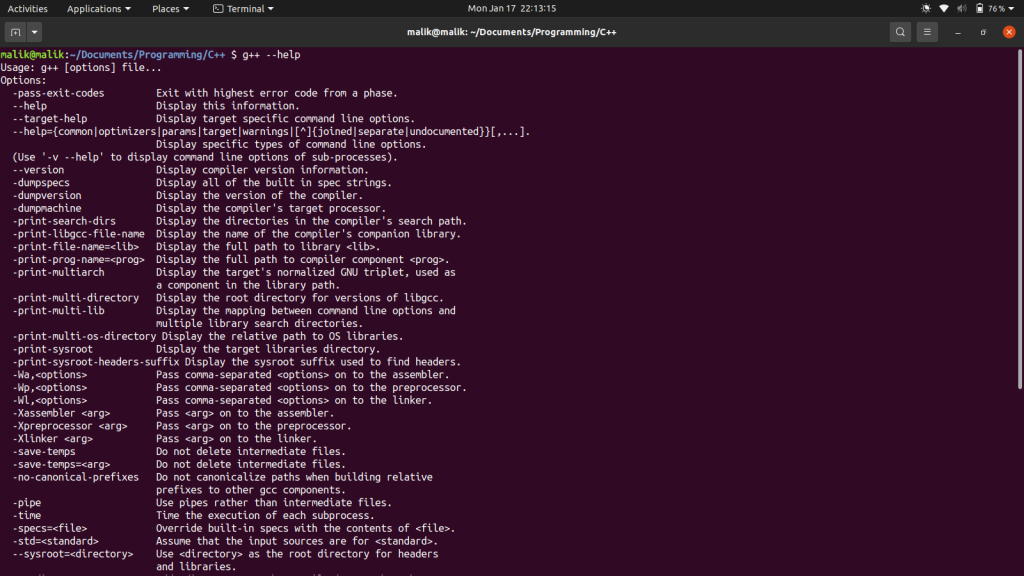
The most commonly used flags are:
- -o: This flag is used for specifying the output file for compilation
- -g: This flag is passed for debugging purposes, it allows gdb to access the symbols and variables used in the program.
- -Wall: It is used to enable the warnings while compiling, this is very useful for developing generic and production grade softwares.
- –time: This lists the time taken by the execution of each subprocess during the compilation. This one is very useful while working with large codes and Competitive Programmmers
Sample Code for Demonstration
Let’s understand the topic with a demo program that we can visualize and use different flags on while compiling. We’ll create a simple Binary Search program and build and run it directly through the terminal.
#include <iostream>
#include <vector>
using namespace std;
int iterations_counter = 0;
int binary_search(vector <int> arr, int s, int e, int key)
{
//base case
iterations_counter++;
if(s>e)
return -1;
int mid = (s+e)/2;
int index = -1;
if(arr[mid] == key)
index = mid;
else if(arr[mid] > key)
index = binary_search(arr, s, mid-1, key);
else
index = binary_search(arr, mid+1, e, key);
return index;
}
int main()
{
cout << "Enter the elements of the vector(Press -1 to stop)" << endl;
vector <int> arr;
while(true)
{
int n;
cin >> n;
if(n == -1)
break;
arr.push_back(n);
}
cout << "Enter the key" << endl;
int key;
cin>>key;
cout<<"Index at which the key is found(0 based indexing, -1 indicates that the key is not present in the vector): "<<binary_search(arr,0, arr.size() - 1, key)<<endl;
cout<<"Total Number of iterations required: "<<iterations_counter<<endl;
}
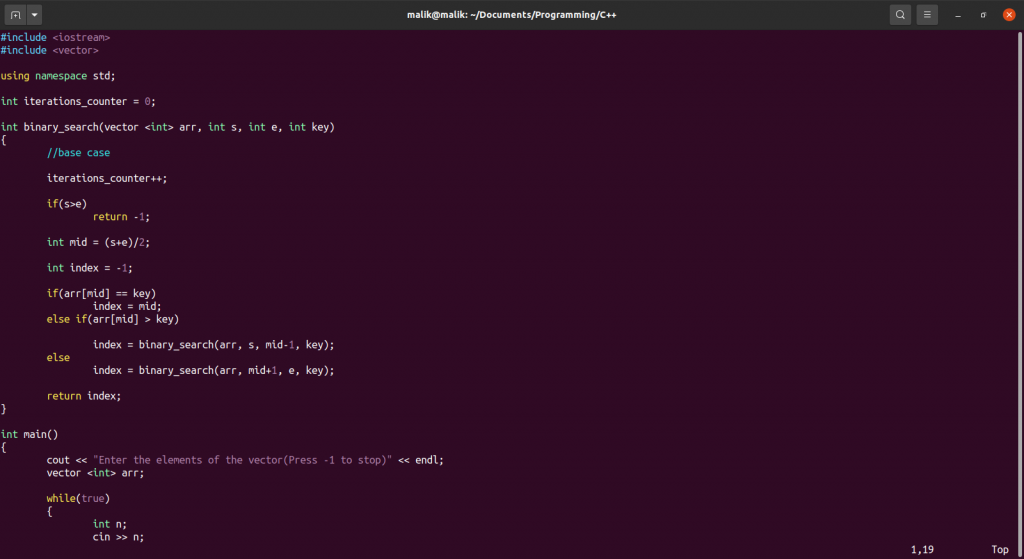
Compiling And Running The Code In The Terminal
Now we’ll see how to compile the code using the Terminal and Execute it.
Note: We can use gcc compiler as well but it can only compile C programs, while g++ can compile C as well as C++ programs. Hence in this article, we are using g++ compiler.
To compile the code using g++ compiler, you need to run the following commands:
1. This one is the most basic one to compile the code and generate an executable file.
g++ "your_code_file_path_without_quotes" -o "your_output_file_path_without_quotes"
2. Let’s compile the code using “-Wall
” flag
g++ "your_code_file_path_without_quotes" -o "your_output_file_path_without_quotes" -Wall
3. Using the “-g
” flag
g++ "your_code_file_path_without_quotes" -o "your_output_file_path_without_quotes" -g
4. Using the “–time” flag
g++ "your_code_file_path_without_quotes" -o "your_output_file_path_without_quotes" --time
5. Using multiple flags at once
g++ "your_code_file_path_without_quotes" -o "your_output_file_path_without_quotes" -g --time -Wall
Note:
- Linux and Mac users are suggested to name the output_file as(without quotes): “some_file_name_without_any_file_type_extensions”.
- Windows users should use “.exe” file extension after their output_file names
Now to run the compiled code we’ll use the executable file that was generated by the compiler, using the following command
1. For Linux and Mac users
./your_output_file_path_without_quotes
2. For Windows users
your_output_file_name_that_ends_with_(.exe)
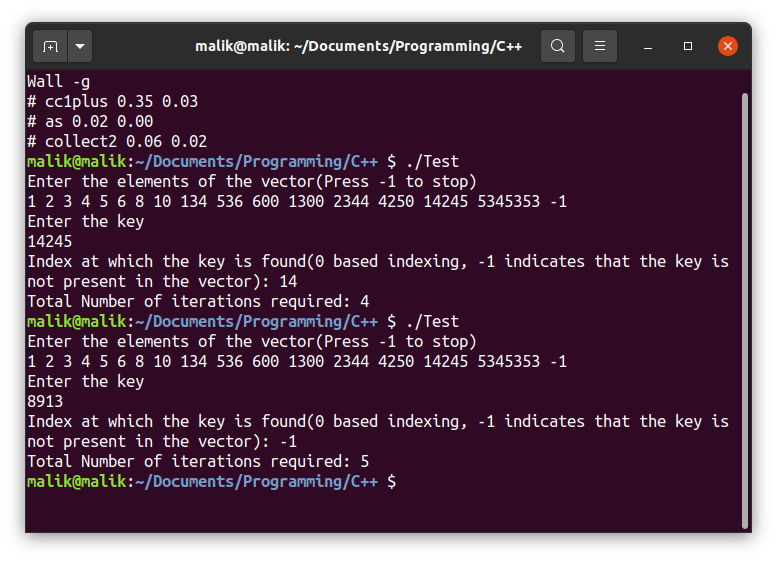
Conclusion
In this article we learned the meaning and uses of different g++ flags, we also learned how to compile and run our C/C++ program in different Operating Systems by going through a simple C++ Binary Search program.
References
To learn more about C++ and g++, you can visit the following sites
- https://gcc.gnu.org/
- https://stackoverflow.com/questions/37868265/run-c-program-in-terminal
- https://en.cppreference.com/w/